Leaflet Day 11 - Plugins
At its core, Leaflet is designed to be lightweight. That being said, there are hundreds of third-party plugins available to extend and enhance the functionality of your web maps.
Today we’ll illustrate adding a plugin to our map from Day 6.
The L.Control.ZoomBar
plugin adds a custom zoom control that allows us to draw a box around the area we want to zoom to, as well as adding a Home
button to return to the initial map view.
Installing the Plugin
The install procedure for a Leaflet plugin varies. Some are installed using npm
, others by manually placing files. For the ZoomBar plugin the install is a manual process.
The plugin and instructions can be found at https://github.com/elrobis/L.Control.ZoomBar. After downloading the files and placing them in the appropriate location(s), we add the following to the head of our HTML file:
<link rel="stylesheet" type="text/css" href="/stylesheets/L.Control.ZoomBar.css"/>
<script type="text/javascript" src="/js/L.Control.ZoomBar.js"></script>
The images for the ZoomBar plugin must be installed in the same location as other Leaflet images, in our case:
static/stylesheets/images
Setting Up the Control
Next we need to make a few modifications to our JavaScript file. The declaration of the map is changed to:
var map = L.map(document.getElementById('mapDIV'), {
center: [63.2, -145.2],
zoom: 7,
zoomControl: false
});
We changed the center of the map a bit, as well as the zoom level so we start out with a broader view. The zoomControl: false
option disables the default zoom control so we can use the ZoomBar.
Now all we need to do is add the ZoomBar at the end of the JavaScript code:
// Add the ZoomBar control..
var zoom_bar = new L.Control.ZoomBar({position: 'topleft'}).addTo(map);
Notice we set the position to the top left corner of the map. By default it will reside there, but this illustrates the option needed to set the location of the control. For example, if we wanted it in the top right corner, we would use:
var zoom_bar = new L.Control.ZoomBar({position: 'topright'}).addTo(map);
With those few changes, we get the new zoom control in the upper left corner:
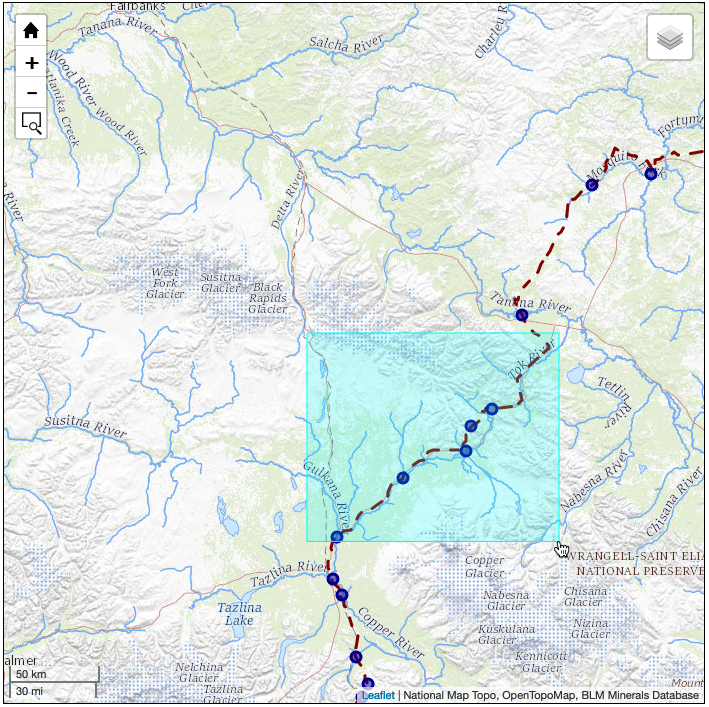
Clicking on the zoom to area (bottom) button allows us to draw a zoom box and zoom to it.
The home button returns us to the initial view.
Note: on small screens (i.e. mobile) the zoom to area button isn’t displayed because of the device’s pinch zooming feature. If you don’t see the bottom button, that’s why.
Take a look at the Leaflet Plugins page to see what else you might find useful.
You can view the map live here.
To view the complete source code, view the source of the leaflet_day11.html
in your browser, then click on the /code/leaflet/leaflet_day11.js
link.