Leaflet Day 10 - Adding a Link to a Popup
In this post we’ll add a link to the towns popup that will display the satellite view on Google Maps.
The API for working with Google Maps URLs can be found here: https://developers.google.com/maps/documentation/urls/guide.
To add a link to the town name in the popup, we modify the JavaScript code that creates the towns layer:
|
|
Lines 11 and 12 setup the URL. When constructed in the onEachFeature
loop, an example looks like this:
This URL will cause the map to center the view on the given coordinates (Valdez) with a zoom level of 10. The
basemap=satellite
parameter sets the map to a satellite view. Other options are roadmap
(the
default) and terrain
.
In lines 13–14 the link is built using the base URL and the coordinate data from our layer. The Elevation and Lat/Lon portion of the popup definition remain unchanged.
Line 18 is commented out to make the popup stay visible when the mouse is moved. This allows us to
click on the link. The popup remains until another receives the mouseover
event or you close it by
clicking on the “x”.
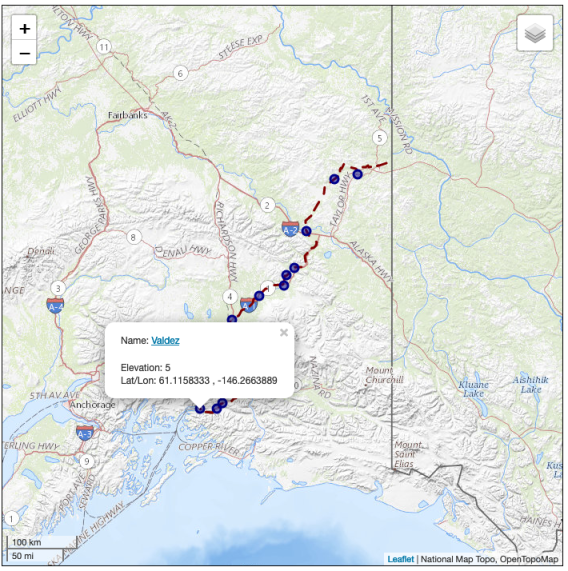
Give it a try by replacing the basemap with terrain or roadmap.
You can view the map live here.
To view the complete source code, view the source of the leaflet_day10.html
in your browser, then click on the /code/leaflet/leaflet_day10.js
link.