Leaflet Day 9 - Calculating Distance with Turf.js
Today we’re going to use Turf.js to calculate the distance between any two points along the trail. Turf.js is billed as providing “Advanced geospatial analysis for browsers and Node.js.”
The distance calculated is a straight line (“as the crow flies”) distance rather than actual trail miles.
Including Turf.js
To calculate the distance we need to include Turf.js
. Rather than install it locally, just add this line to the head of your HTML:
<script src='https://npmcdn.com/@turf/turf/turf.min.js'></script>
Turf.js comes as a single JavaScript file and is quite large. If you plan to use it regularly, consider making a custom build that contains only those features you need. You can find info on how to do that in the Leaflet Cookbook and on the Turf.js website.
Setting up the HTML
We need to add two dropdown (select) boxes: one for the “from” location, and the other for the “to” location. We also create a “calculate” button, and a div to contain the result:
Calculate distance from:
<select id='fromBox'>
</select>
to:
<select id='toBox'>
</select>
<input type="button" id="calculate" value="Calculate">
<div id="distanceResult"></div>
Calculating and Displaying the Distance
First we setup the two dropdown boxes with the list of towns.
To calculate the distance, we get the from and to locations from the dropdown boxes then pass the
coordinates as turf.point
objects to the Turf.js distance function.
|
|
Lines 1–16 populate the dropdown (select) boxes using the data in the trail_stops
GeoJSON.
Line 18 sets up the calculate button to call the calculateDistance
function (lines 20–33).
In lines 21-26, we get the from/to towns and using the featuremap
, get the latitude and longitude
to create two turf.point
objects. Notice turf.point
is created by specifying the longtiude (x
value) first.
Line 27 calculates the distance and line 28 just logs it to the console.
Line 29 converts from kilometers to miles.
Lines 31–32 set the text for the distanceResult
div.
Calculating a distance gives us the result in both kilometers and miles:
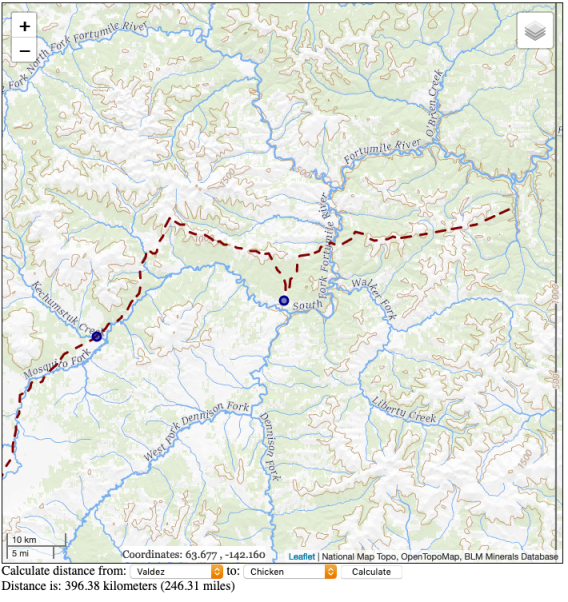
You can view the map live here.
To view the complete source code, view the source of the leaflet_day9.html
in your browser, then click on the /code/leaflet/leaflet_d9.js
link.